UbuntuにTypeScriptをインストール
JavaScriptに型付やクラスの機能を追加したTypeScriptと言うプログラミング言語を使ってみました。 初めて使う素人がインストールして最小限のプログラムを実行するまでの操作メモです。
OSは"Ubuntu 20.04.2"です。
Node.jsをインストールする
TypeScriptは初めて使用するので無難に公式ページを参考にしながら進めます。
https://www.typescriptlang.org/download
今回はnpmパッケージ管理システムを使用してインストールし、プログラムの動作確認もするため先に"Node.js"をインストールしておきます。
https://github.com/nodesource/distributions/blob/master/README.md
以下のバージョンでこれから進めていきます。
$ npm -v
6.14.15
$ node -v
v14.17.6
公式ページではTypeScriptのインストールは、プロジェクト毎にするとバージョン管理しやすいとあります。素直にプロジェクトを作成し、そこにインストールすることにします。
# プロジェクトディレクトリを作成し
$ mkdir workts
# 移動します
$ cd workts
# プロジェクトを初期化
# 途中でいろいろたずねられますが全て何も入力せず[Enter]キーで進めます
$ npm init
package name: (workts)
version: (1.0.0)
description:
entry point: (index.js)
test command:
git repository:
keywords:
author:
license: (ISC)
About to write to /home/izumi/workts/package.json:
{
"name": "workts",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC"
}
Is this OK? (yes)
# 最新のTypeScriptをカレントディレクトリにインストール
$ npm install typescript --save-dev
インストールされたバージョンを確認してみます。
npx
はローカルにインストールしたパッケージを簡単に実行できるコマンドらしいです。
よく分かっていませんがとりあえず進めます。
$ npx tsc --version
Version 4.4.3
バージョンを指定してインストールする場合は、@の後にバージョンを指定します。
npm install typescript@1.0.0 --save-dev
TypeScriptプログラムのコンパイルと実行
とりあえず実行するためのTypeScriptコードを作成します。
test.ts
const greets = (name: string) => {
console.log(`Hello ${name}`);
};
greets('(=^・・^=)');
そのままでは実行することができないのでnpx tsc ファイル名
コマンドで、“test.ts"ファイルをコンパイルします。
$ npx tsc test.ts
コンパイルが成功しtest.js
というJavaScriptファイルが出力されました。
$ ls -l
合計 28
drwxrwxr-x 4 izumi izumi 4096 9月 20 14:34 node_modules
-rw-rw-r-- 1 izumi izumi 384 9月 20 14:34 package-lock.json
-rw-rw-r-- 1 izumi izumi 257 9月 20 14:34 package.json
-rw-rw-r-- 1 izumi izumi 92 9月 22 20:40 test.js
-rw-rw-r-- 1 izumi izumi 96 9月 22 20:34 test.ts
さっそくNode.jsを使って実行します。
$ node test.js
Hello (=^・・^=)
設定ファイルを使ったTypeScriptの実行
設定ファイル"tsconfig.json"を使用すれば複雑なTypeScriptアプリも作れるようです。
とりあえずnpx tsc --init
コマンドでデフォルトの設定ファイルを作成しました。
$ npx tsc --init
message TS6071: Successfully created a tsconfig.json file.
“tsconfig.json"ファイルが作成されました。
TypeScriptのコードを書いていきます。 “Hello World!“を表示するメソッドと呼び出すコードを2つのファイルに分けて作成します。
index.ts
import { hello } from './hello';
hello('🌏',' ');
hello.ts
// HELLO WORLD! と出力する
export const hello = (front: string, back: string) => {
const data: Array<string> = [
"121141141524111112223214332",
"12111414141213111111121112111412122",
"4132141412131111111211321412122",
"1211141414121311111112111112141214",
"121141414225111322121141332"];
data.forEach(line => {
let buffer: string = "";
// 文字列をスプレッド構文で1文字の配列へ変換、更に数値へ
let lineArray = [...line].map(n => Number(n));
const max = lineArray.length;
for (let i = 0; i < max; i++) {
// 交互に文字をbufferに追加する
let typeChar: string = front;
if (i % 2 != 0) {
typeChar = back;
}
for (let j = 0; j < lineArray[i]; j++) {
buffer += typeChar;
}
}
console.log(buffer);
});
};
設定ファイルに記述された内容でコンパイルされるのでnpx tsc
コマンドをオプションを指定せずに実行します。
$ npx tsc
hello.ts:12:29 - error TS2461: Type 'string' is not an array type.
12 let lineArray = [...line].map(n => Number(n));
~~~~
Found 1 error.
いきなりエラーになりました。
文字列を1文字ずつ処理するためにスプレッド構文で配列に分解したいのですが、この方法が使えるのは"es6"からでした。設定ファイル"tsconfig.json"を見てみると"es5"になっていたので"es6"に変更します。
tsconfig.json
・・・中略・・・
/* Language and Environment */
"target": "es5", /* Set the JavaScript language version for emitted JavaScript and include compatible library declarations. */
・・・中略・・・
↓以下のように変更
"target": "es6", /* Set the JavaScript language version for emitted JavaScript and include compatible library declarations. */
再びコンパイルします。
$ npx tsc
“hello.js"と"hello.js"が出力されています。
$ ls
hello.js hello.js node_modules package.json test.ts
hello.ts index.ts package-lock.json test.js tsconfig.json
Node.jsで実行すると"Wello World!“と表示されました。
$ node index.js
🌏 🌏 🌏🌏🌏🌏 🌏 🌏 🌏🌏 🌏 🌏 🌏 🌏🌏 🌏🌏🌏 🌏 🌏🌏🌏 🌏🌏
🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏🌏
🌏🌏🌏🌏 🌏🌏🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏🌏🌏 🌏 🌏 🌏 🌏🌏
🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏 🌏
🌏 🌏 🌏🌏🌏🌏 🌏🌏🌏🌏 🌏🌏🌏🌏 🌏🌏 🌏 🌏 🌏🌏 🌏 🌏 🌏🌏🌏🌏 🌏🌏🌏 🌏🌏
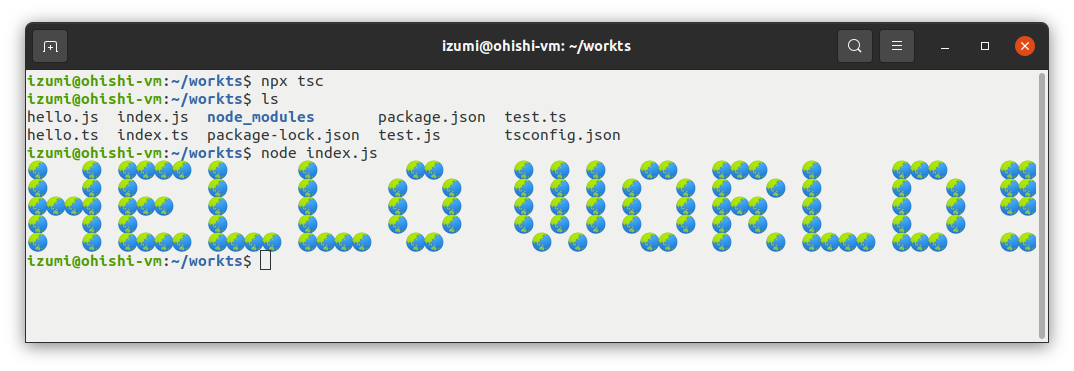